Python is one of the most popular programming languages, used in web development, data analysis, automation, etc. It is an interpreted language. This means that instead of converting the entire program to a lower-level language at once, it translates each code line, one at a time. While scanning the code, the Python interpreter may find an error in a line that can be a syntax error. Missing quotation marks, missing brackets, etc. can constitute improper syntax.
In this article, we shall understand what is EOL while scanning string literal Python and fixes for it.
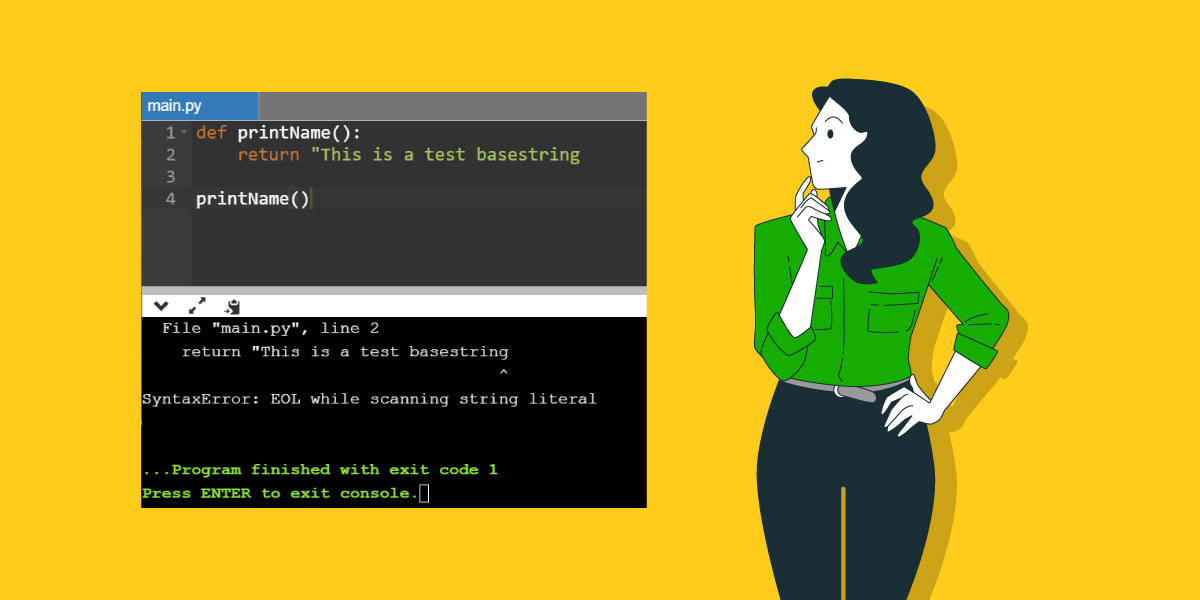
What is the ‘Python error EOL while scanning’?
EOL full form in Python is “end of line”. The EOL Python meaning is that the the Python interpreter has come to the line’s end or EOL while scanning a string literal. Both double and single quotation marks are used while mentioning string literal in your code. When someone does not mention quotation marks, Python interpreter will reach the end of line or EOL while scanning the last character of the string. For example,
# Storing string value
st = “Example of string literal
# Printing the string st
print(st)
You get the below mentioned results when you run the code.
File “EOL.py”, line 2
st = “Example of string literal
SyntaxError EOL while scanning string literal
Reasons for the error and their solutions:
Users can encounter this EOL scanning string literal Python error due to four reasons.
-
Missing an ending quotation mark
Python interpreter will raise a syntax error when incorrect syntax is used. If quotation marks are missing in a string literal, an EOL Python error will occur.
# Ending quotation mark missing
st = “Example of string literal
# Printing the string st
print(st)
The fix to this problem is to follow proper syntax rules defined by Python language and use quotation marks while using a string literal.
# Ending quotation mark missing
st = “Example of string literal”
# Printing the string st
print(st)
-
Incorrect ending quotation mark
In Python, you can use “ ” and ‘ ’ to keep the string literals enclosed. Interchanging them or using them simultaneously will generate the error.
# Ending quotation mark incorrect
st = ‘Example of string literal”
# Printing the string st
print(st)
To fix this, just use single or double quotation marks. Don’t mix them up.
# Ending quotation mark incorrect
st = “Example of string literal”
# Printing the string st
print(st)
-
Stretching string constant to multiple lines
Sometimes programmers use multiple lines while writing a string. Unlike Java and C++, a new line in Python means the end of string statement. When Python interpreter jumps to next line, an error is caused.
# Stretched string
st = “Example of string literal
Next line extension”
# Printing the string st
print(st)
You can fix this by using \n or newline character.
# Stretched string
st = “Example of string literal \n Next line extension”
# Printing the string st
print(st)
Triple quotes can also be used to address this error.
-
Backslash before an ending quotation mark
Using “\” can escape the string and Python interpreter will consider it as one character.
# backlash
st = “\home\User\Pictures\”
# Printing the string st
print(st)
It can be fixed by using “\\” instead of “\”.
# backlash
st = “\\home\\User\\Pictures\\”
# Printing the string st
print(st)
Conclusion
We have covered the causes as well as the solutions for the EOL Python error. Programmers should pay attention to the syntax to avoid this error.